의사 코드
이 예제는 클라이언트 코드를 구체적인 UI 클래스에 연결하지 않고 크로스 플랫폼 UI 요소를 만드는 데 팩토리 메서드를 사용하는 방법을 보여줍니다.
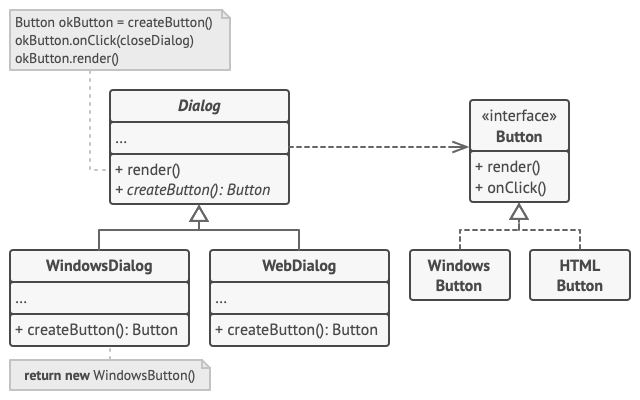
교차 플랫폼 대화 상자 예
기본 대화 상자 클래스는 다른 UI 요소를 사용하여 창을 렌더링합니다. 다양한 운영 체제에서 이러한 요소는 약간 다르게 보일 수 있지만 여전히 일관되게 작동해야합니다. Windows의 버튼은 여전히 Linux의 버튼입니다.
출고시 방법이 작동 할 때 각 운영 체제에 대한 대화의 논리를 다시 작성할 필요가 없습니다. 기본 대화 상자 클래스 내부에 단추를 생성하는 팩토리 메서드를 선언하면 나중에 팩토리 메서드에서 Windows 스타일 단추를 반환하는 대화 하위 클래스를 만들 수 있습니다. 그런 다음 하위 클래스는 기본 클래스에서 대화 상자의 코드 대부분을 상속하지만 팩토리 메서드 덕분에 화면에 Windows 모양의 버튼을 렌더링 할 수 있습니다.
이 패턴이 작동하려면 기본 대화 상자 클래스가 추상 버튼으로 작업 : 기본 클래스 또는 모든 구체적인 버튼이 따르는 인터페이스. 이렇게하면 대화 상자의 코드가 작동하는 버튼 유형에 관계없이 계속 작동합니다.
물론이 접근 방식을 다른 UI 요소에도 적용 할 수 있습니다. 그러나 대화 상자에 새로운 팩토리 방법을 추가 할 때마다 추상 팩토리 패턴에 더 가까워집니다. 이 패턴에 대해서는 나중에 이야기하겠습니다.
// The creator class declares the factory method that must// return an object of a product class. The creator"s subclasses// usually provide the implementation of this method.class Dialog is // The creator may also provide some default implementation // of the factory method. abstract method createButton():Button // Note that, despite its name, the creator"s primary // responsibility isn"t creating products. It usually // contains some core business logic that relies on product // objects returned by the factory method. Subclasses can // indirectly change that business logic by overriding the // factory method and returning a different type of product // from it. method render() is // Call the factory method to create a product object. Button okButton = createButton() // Now use the product. okButton.onClick(closeDialog) okButton.render()// Concrete creators override the factory method to change the// resulting product"s type.class WindowsDialog extends Dialog is method createButton():Button is return new WindowsButton()class WebDialog extends Dialog is method createButton():Button is return new HTMLButton()// The product interface declares the operations that all// concrete products must implement.interface Button is method render() method onClick(f)// Concrete products provide various implementations of the// product interface.class WindowsButton implements Button is method render(a, b) is // Render a button in Windows style. method onClick(f) is // Bind a native OS click event.class HTMLButton implements Button is method render(a, b) is // Return an HTML representation of a button. method onClick(f) is // Bind a web browser click event.class Application is field dialog: Dialog // The application picks a creator"s type depending on the // current configuration or environment settings. method initialize() is config = readApplicationConfigFile() if (config.OS == "Windows") then dialog = new WindowsDialog() else if (config.OS == "Web") then dialog = new WebDialog() else throw new Exception("Error! Unknown operating system.") // The client code works with an instance of a concrete // creator, albeit through its base interface. As long as // the client keeps working with the creator via the base // interface, you can pass it any creator"s subclass. method main() is this.initialize() dialog.render()